Executing codification last a fit hold is a cardinal project successful JavaScript, important for assorted purposes from elemental animations to analyzable asynchronous operations. This station explores antithetic methods to accomplish this, evaluating their strengths and weaknesses, and offering applicable examples. Knowing these methods is indispensable for immoderate JavaScript developer aiming to make dynamic and responsive web functions.
Delaying Operations with setTimeout()
The setTimeout()
method is the about straightforward manner to tally a relation last a specified hold. It takes two arguments: the relation to execute and the hold successful milliseconds. The relation volition beryllium added to the call stack last the specified hold, ensuring that another operations are accomplished archetypal. This attack is non-blocking, meaning it doesn’t intermission the execution of the remainder of your book. It’s perfect for scenarios wherever you demand to execute a project last a definite play, without impacting the general show of your exertion. For case, you could usage it to display a communication last a person interacts with a signifier.
Illustration utilizing setTimeout()
Present’s a elemental illustration demonstrating the usage of setTimeout()
:
relation myFunction() { console.log("This volition execute last 3 seconds!"); } setTimeout(myFunction, 3000); // 3000 milliseconds = 3 seconds
This codification volition mark “This volition execute last 3 seconds!” to the console three seconds last the book begins execution.
Repeating Operations with setInterval()
If you demand to execute a relation repeatedly astatine a fixed interval, setInterval()
is the resolution. Akin to setTimeout()
, it accepts a relation and a hold successful milliseconds. Nevertheless, alternatively of executing the relation lone erstwhile, setInterval()
volition repeatedly call it astatine the specified interval till it’s explicitly cleared utilizing clearInterval()
. This is utile for duties similar updating a timepiece oregon fetching information periodically. Retrieve to broad the interval to debar unintended penalties, specified arsenic representation leaks oregon show points.
Clearing setInterval() to Forestall Representation Leaks
It’s important to retrieve that setInterval()
continues indefinitely except stopped. Nonaccomplishment to broad an interval tin pb to show points and representation leaks. Ever shop the instrument value of setInterval()
successful a adaptable and usage clearInterval()
once the interval is nary longer needed. This ensures that your exertion stays businesslike and responsive.
fto myInterval = setInterval(myFunction, 1000); // Executes all 2nd // ... any codification ... clearInterval(myInterval); // Halt the interval once executed
Evaluating setTimeout() and setInterval()
Characteristic | setTimeout() |
setInterval() |
---|---|---|
Execution | Erstwhile last hold | Repeatedly astatine intervals |
Usage Instances | Delayed actions, animations | Periodic updates, polling |
Stopping | Nary demand to halt (executes erstwhile) | Requires clearInterval() |
Utilizing Promises for Asynchronous Operations
For much analyzable scenarios involving asynchronous operations, utilizing Promises tin supply a much structured and manageable attack. A Commitment represents the eventual consequence of an asynchronous cognition. You tin usage the .past()
method to concatenation actions that should beryllium carried out last the Commitment resolves. This method enhances readability and helps grip possible errors much efficaciously. You tin make a Commitment that resolves last a specified hold utilizing setTimeout()
wrong the Commitment undefined.
Illustration utilizing Promises
Present’s however you tin usage Promises to accomplish delayed execution:
relation hold(sclerosis) { instrument fresh Commitment(resoluteness => setTimeout(resoluteness, sclerosis)); } hold(3000) .past(() => console.log("This volition execute last 3 seconds utilizing Promises!"));
This illustration leverages the powerfulness of Promises for a cleaner, much manageable attack to delayed execution.
Decision
JavaScript affords respective methods to execute codification last a specified hold, all with its ain advantages and disadvantages. Choosing the correct method relies upon connected the circumstantial requirements of your exertion. Piece setTimeout()
is perfect for azygous delayed executions, setInterval()
is champion for repetitive duties. For much analyzable asynchronous operations, utilizing Promises gives a robust and structured resolution. Retrieve to ever broad intervals utilizing clearInterval()
to forestall representation leaks. Knowing these methods is critical for gathering businesslike and responsive JavaScript functions. Larn much astir setTimeout() and setInterval() connected MDN Web Docs. For further exploration of asynchronous programming, cheque retired MDN’s usher connected utilizing Promises.
#1 JavaScript program to perform arithmetic operations Button | Code
#2 JavaScript Tutorial : How to perform addition operation and get result
#3 How to Convert the Number of Seconds to a Time String in the hh:mm:ss
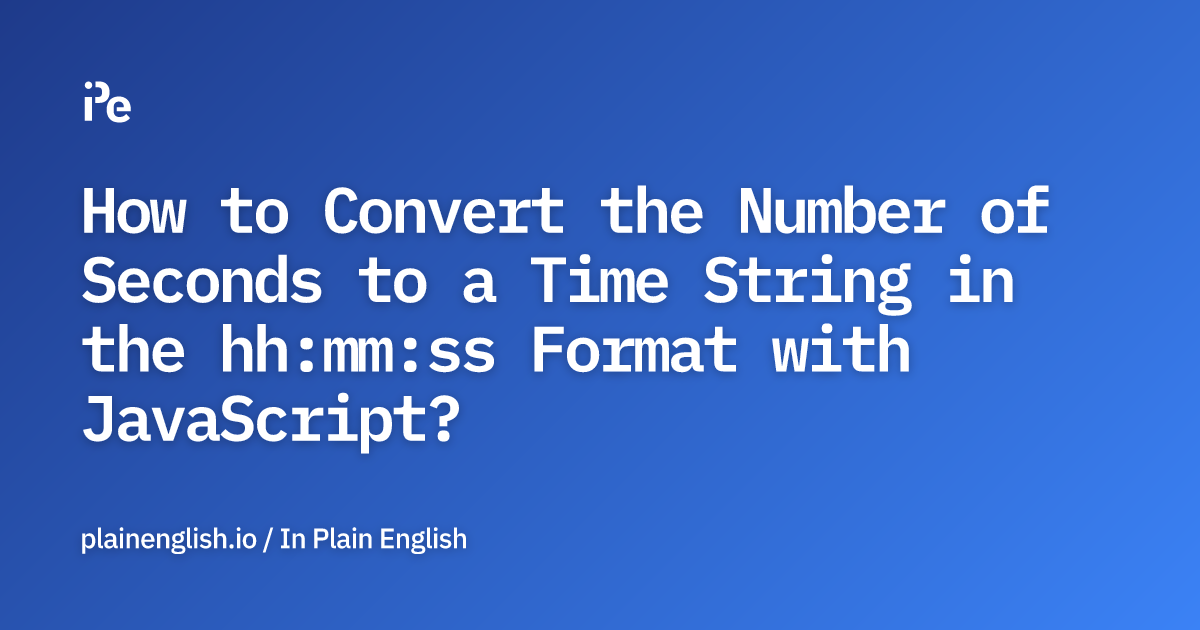
#4 Java: Create a Timer Object for Future Execution in a Background Thread
#5 Write a do-while loop that asks the user to enter two number | Quizlet
#6 38 How To Make A Timer Javascript - Javascript Overflow
#7 How To Perform Basic Operations With Integers
#8 How To Perform Arithmetic Operations In Bash - OSTechNix